Learn Rust by debugging Rust
Gaurav Kamathe
Fri, 07/29/2022 – 03:00
In my previous article about rustup, I showed you how to install the Rust toolchain. Well, what good is the toolchain if you won’t be using it to get more hands-on with Rust? Learning any language involves reading existing code and writing a lot of sample programs. That’s a good way to become proficient in a language. However, there’s a third way: debugging code.
Learning through debugging involves trying to compile a pre-written (buggy) sample program, understanding the errors generated by the compiler, fixing the sample code, and then re-compiling it. Repeat that process until the code successfully compiles and runs.
Rustlings is an open source project by the Rust team that helps you learn Rust through the process of debugging. It also provides you with a lot of hints along the way. If you’re a beginner to Rust and have either started or completed reading the Rust book, then rustlings is the ideal next step. Rustlings helps you apply what you’ve learned from the book, and move to working on bigger projects.
Installing rustlings
I’m using (and recommend) a Fedora machine to try rustlings, but any Linux distribution works. To install rustlings, you must download and run its install script. It’s recommended that you do this as a normal user (not root) without any special privileges.
Remember, for you to be able to use rustlings, you need the Rust toolchain available on your system. If you don’t have that already, please refer to my article on rustup.
Once you’re ready, download the installation script:
$ curl -L https://raw.githubusercontent.com/rust-lang/rustlings/main/install.sh > rustlings_install.sh
$ file rustlings_install.sh
rustlings_install.sh: Bourne-Again shell script, ASCII text executable
Inspect the script to learn what it does:
$ less rustlings_install.sh
And then run it to install:
$ bash rustlings_install.sh
[...]
Installing /home/tux/.cargo/bin/rustlings
Installed package `rustlings v4.8.0 (/home/tux/rustlings)` (executable `rustlings`)
All done!
Run ‘rustlings’ to get started.
Rustlings exercises
The installation provides you with the rustlings
command. Run it along with the --help
flag to see what options are available.
$ rustlings --help
The installation script also clones the rustlings Git repository, and installs all the dependencies required to run the sample programs. You can view the sample programs within the exercises directory under rustlings
:
$ cd rustlings
$ pwd
/home/tux/rustlings
$ ls
AUTHORS.md Cargo.toml CONTRIBUTING.md info.toml install.sh README.md target Cargo.lock CHANGELOG.md exercises install.ps1 LICENSE src tests
$ ls -m exercises/
advanced_errors, clippy, collections, conversions, enums, error_handling, functions, generics, if, intro, macros, mod.rs,
modules, move_semantics, option, primitive_types, quiz1.rs, quiz2.rs, quiz3.rs, quiz4.rs, README.md,
standard_library_types, strings, structs, tests, threads, traits, variables
List all exercises from the command line
The rustlings
command provides you with a list
command which displays each sample program, its complete path, and the status (which defaults to pending.)
$ rustlings list
Name Path Status
intro1 exercises/intro/intro1.rs Pending
intro2 exercises/intro/intro2.rs Pending
variables1 exercises/variables/variables1.rs Pending
variables2 exercises/variables/variables2.rs Pending
variables3 exercises/variables/variables3.rs Pending
[...]
Near the end of the output, you’re given a progress report so you can track your work.
Progress: You completed 0 / 84 exercises (0.00 %).
View sample programs
The rustings list
command shows you what programs are available, so at any time you can view the code of those programs by copying the complete paths into your terminal as an argument for the cat or less commands:
$ cat exercises/intro/intro1.rs
Verify your programs
Now you can start debugging programs. You can do that using the verify
command. Notice that rustlings chooses the first program in the list (intro1.rs
), tries to compile it, and succeeds:
$ rustlings verify
Progress: [-----------------------------------] 0/84
✅ Successfully ran exercises/intro/intro1.rs!
You can keep working on this exercise,
or jump into the next one by removing the `I AM NOT DONE` comment:
6 | // Execute the command `rustlings hint intro1` for a hint.
7 |
8 | // I AM NOT DONE
9 |
As you can see from the output, even though the sample code compiles, there’s work yet to be done. Each sample program has the following comment in its source file:
$ grep "NOT DONE" exercises/intro/intro1.rs
// I AM NOT DONE
Although the compilation of the first program worked fine, rustlings won’t move to the next program until you remove the I AM NOT DONE
comment.
Moving to the next exercise
Once you have removed the comment from intro1.rs
, you can move to the next exercise by running the rustlings verify
command again. This time, you may notice that rustlings tries to compile the next program (intro2.rs
) in the series, but runs into an error. You’re expected to debug that issue, fix it, and then move forward. This is a critical step, allowing you to understand why Rust says a program has bugs.
$ rustlings verify
Progress: [>------------------------] 1/84
⚠️ Compiling of exercises/intro/intro2.rs failed! Please try again. Here's the output:
error: 1 positional argument in format string, but no arguments were given
--> exercises/intro/intro2.rs:8:21
|
8 | println!("Hello {}!");
| ^^
error: aborting due to previous error
Getting a hint
Rustlings has a handy hint
argument, which tells you exactly what’s wrong with the sample program, and how to fix it. You can think of it as an add-on help option, in addition to what the compiler error message tells you.
$ rustlings hint intro2
Add an argument after the format string.
Based on the above hint, fixing the program is easy. All you need to do is add an additional argument to the println
statement. This diff should help you understand the changes:
println!("Hello {}!", "world");
---
> println!("Hello {}!");
Once you make that change, and removed the NOT DONE
comment from the source code, you can run rustlings verify
again to compile and run the code.
$ rustlings verify
Progress: [>-------------------------------------] 1/84
✅ Successfully ran exercises/intro/intro2.rs!
Track progress
You aren’t going to finish all of the exercises in a day, and it’s easy to lose track of where you left off. You can run the list
command to see the status of your work.
$ rustlings list
Name Path Status
intro1 exercises/intro/intro1.rs Done
intro2 exercises/intro/intro2.rs Done
variables1 exercises/variables/variables1.rs Pending
variables2 exercises/variables/variables2.rs Pending
variables3 exercises/variables/variables3.rs Pending
[...]
Run specific exercises
If you don’t want to start from the beginning and skip a few exercises, rustlings allows you to focus on specific exercises using the rustlings run
command. This runs a specific program without requiring the previous lesson to be verified. For example:
$ rustlings run intro2
Hello world!
✅ Successfully ran exercises/intro/intro2.rs
$ rustlings run variables1
Typing exercise names can become tedious, but rustlings provides you with a handy next
command so you can move to the next exercise in the series.
$ rustlings run next
Alternative watch command
If you don’t want to keep typing verify
after each modification you make, you can run the watch
command in a terminal window, and then keep modifying the source code to fix issues. The watch
command detects these modifications, and keeps re-compiling the program to see whether the issue has been fixed.
$ rustlings watch
Learn by debugging
The Rust compiler is known to provide very meaningful error messages, which helps you understand issues in your code. This usually leads to faster debugging. Rustlings is a great way to practice Rust, to get used to reading error messages, and understand the Rust language. Check out the recent features of Rustlings 5.0.0 on GitHub.
[ Practice programming with Rust. Download our Rust cheat sheet. ]
Rustlings is an open source project by the Rust team that helps you learn Rust through the process of debugging.
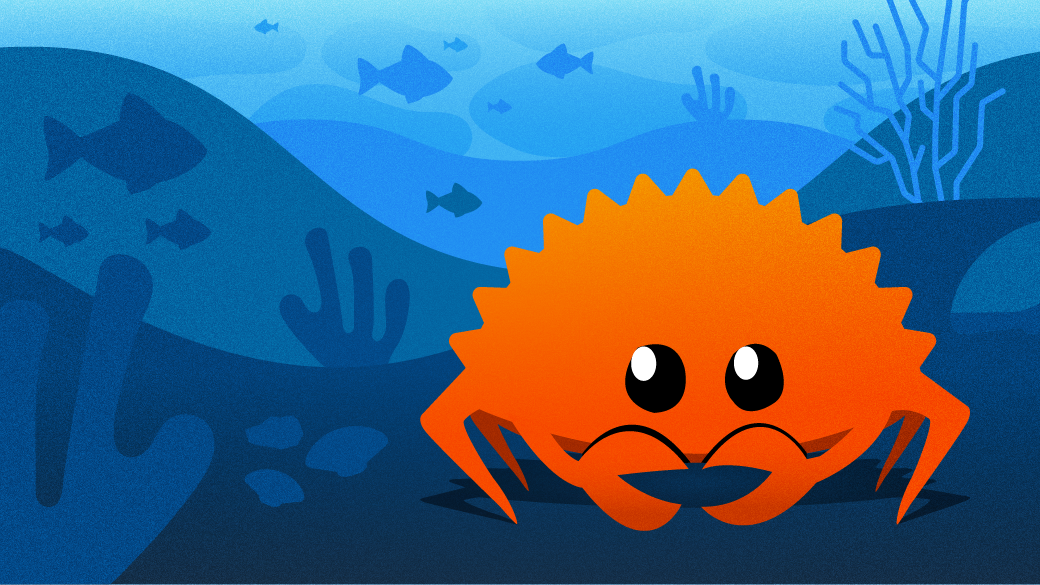
Opensource.com
Powered by WPeMatico